How to write Python extensions in Rust with PyO3
- arpitnearlearn
- Mar 22, 2023
- 3 min read
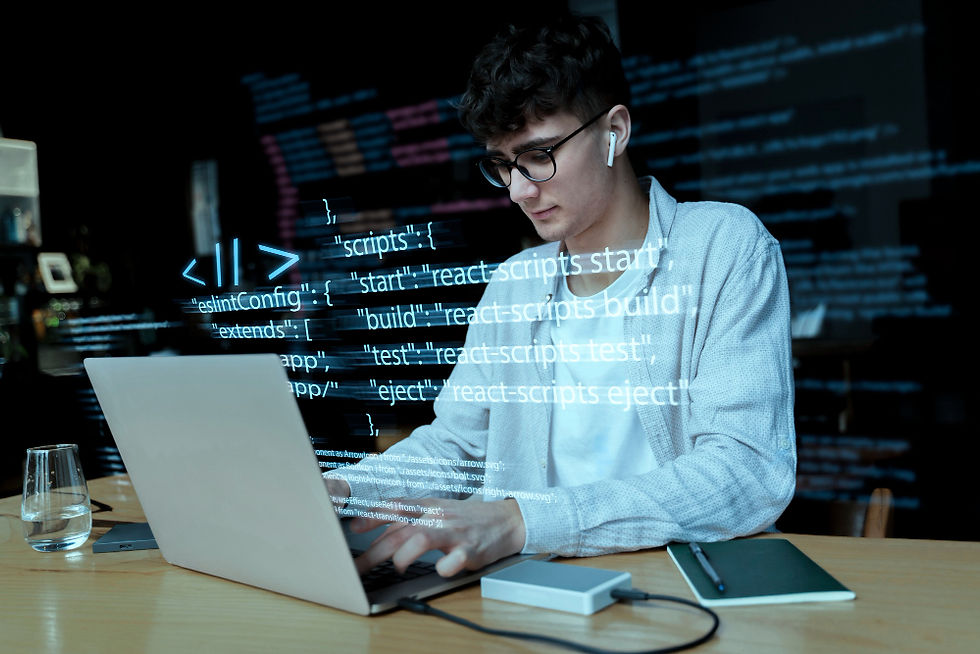
Python is a powerful programming language with a rich ecosystem of libraries and tools. However, sometimes you need to write extensions in other languages to improve performance or access system-level functionality. Rust is a modern systems programming language that provides the safety and performance of a low-level language while still being easy to use. PyO3 is a Rust library that enables you to write Python extensions in Rust. In this article, we'll cover the basics of how to write Python extensions in Rust with PyO3.
Introduction to PyO3
PyO3 is a Rust library that allows you to write Python extensions in Rust. It provides a simple and safe way to interface with Python, allowing you to call Python functions from Rust and vice versa. PyO3 handles all the details of converting between Rust and Python types, making it easy to write high-performance Python extensions in Rust.
Setting up your development environment
Before you can start writing Python extensions in Rust with PyO3, you'll need to set up your development environment. You'll need to install Rust and Python, as well as PyO3 itself. You can install Rust and Python using your operating system's package manager, or download them from their respective websites. To install PyO3, add it to your Rust project's dependencies in the Cargo.toml file.
Writing a simple Python extension in Rust
Once you have your development environment set up, you can start writing your first Python extension in Rust. To do this, you'll need to create a Rust library that exports a C-compatible interface. You can use PyO3 to generate the necessary C code for you, which you can then compile into a shared library that can be loaded by Python. If you're looking for training in python, then you can check out our Python course in Bangalore.
Here's a simple example of a Rust function that adds two numbers:
rust
Copy code
#[pyfunction]
fn add(a: i32, b: i32) -> i32 {
a + b
}
This function uses the pyfunction attribute to tell PyO3 that it should be exported as a Python function. The a and b arguments are passed as i32 types, which PyO3 automatically converts to Python integers. The result is also an i32, which PyO3 converts back to a Python integer.
To compile this function into a Python extension, you'll need to create a lib.rs file that exports it:
scss
Copy code
use pyo3::prelude::*;
#[pymodule]
fn my_module(_py: Python, m: &PyModule) -> PyResult<()> {
m.add_function(wrap_pyfunction!(add, m)?)?;
Ok(())
}
This code uses the pymodule attribute to tell PyO3 that this is a Python module. The add_function method adds our add function to the module, and the wrap_pyfunction macro generates the necessary C code for Python to call the Rust function.
Compiling and using the Python extension
To compile our Python extension, we can use Cargo, Rust's package manager:
css
Copy code
cargo build --release
This will generate a shared library in the target/release directory, which we can load in Python using the ctypes module:
python
Copy code
import ctypes
lib = ctypes.CDLL('target/release/libmy_module.so')
result = lib.add(1, 2)
print(result) # prints 3
This code loads our Rust library and calls the add function with the arguments 1 and 2. The result is returned as a Python integer, which we can print to the console. If you're looking for training in react native, then you can check out our react native course in Bangalore.
Conclusion
In conclusion, PyO3 is a powerful tool that allows developers to write Python extensions in Rust. With its easy-to-use interface and comprehensive documentation, PyO3 offers a simple and efficient way to integrate Rust into your Python code. By using Rust to build your Python extensions, you can take advantage of Rust's performance, safety, and stability while still enjoying the benefits of Python's dynamic nature. Whether you're a seasoned Rust developer or a Python enthusiast looking to optimize your code, PyO3 is an excellent choice for building high-performance Python extensions in Rust.
Comments