How to Run Your Python Code Concurrently Using Threads
- arpitnearlearn
- Mar 24, 2023
- 3 min read
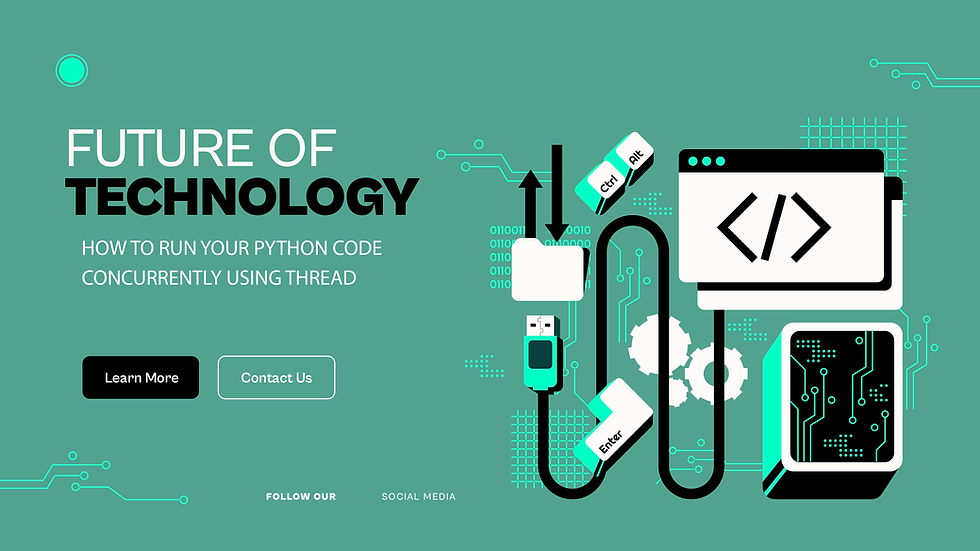
Python is a powerful language that allows developers to create a wide range of applications, from web development to data analysis. However, when it comes to running code concurrently, Python can be a bit limited. One solution to this problem is to use threads. In this article, we will discuss what threads are, how to use them, and some best practices for using them effectively in Python.
What are Threads?
Threads are a way to run multiple tasks concurrently within a single program. A thread is a separate flow of execution within a program, which means that multiple threads can be executed simultaneously. Each thread runs independently and can perform its own tasks, but they share the same memory space.
Threads can be used to improve the performance of a program by allowing it to execute multiple tasks at the same time. This is particularly useful for tasks that are I/O-bound, meaning that they spend a lot of time waiting for input/output operations to complete, such as reading or writing files, making HTTP requests, or querying a database. If you're looking for training in python, then you can check out our Python course in Bangalore.
Using Threads in Python
Python has a built-in module called threading that allows developers to create and manage threads. To use threads in Python, you need to import the threading module and create a new Thread object for each task you want to run concurrently. Here is an example:
ruby
Copy code
import threading
def task1():
# code for task1
def task2():
# code for task2
# create threads
thread1 = threading.Thread(target=task1)
thread2 = threading.Thread(target=task2)
# start threads
thread1.start()
thread2.start()
# wait for threads to finish
thread1.join()
thread2.join()
In this example, we define two functions task1 and task2, which represent the tasks we want to run concurrently. We then create two Thread objects, one for each task, and start them using the start method. Finally, we use the join method to wait for both threads to finish before the program exits.
Best Practices for Using Threads
When using threads, there are a few best practices you should follow to ensure that your program runs smoothly and efficiently:
Avoid shared data: Since threads share the same memory space, it's important to avoid accessing shared data concurrently to prevent race conditions and other synchronization issues. If you need to share data between threads, use thread-safe data structures such as locks, queues, or semaphores.
Use the right number of threads: Creating too many threads can actually slow down your program, as the overhead of managing multiple threads can become significant. Try to find the right balance between the number of threads and the tasks you want to run concurrently.
Prioritise tasks: If you have tasks that are more important than others, you can use thread priorities to ensure that they are executed first. However, be careful not to starve other threads of resources. If you're looking for training in react native, then you can check out our react native course in Bangalore.
Use a thread pool: Creating and managing threads can be expensive, especially if you have many short-lived tasks. Consider using a thread pool to reuse threads and reduce overhead.
Conclusion
Using threads is a powerful way to run multiple tasks concurrently in Python. It can improve the performance of your program, especially for I/O-bound tasks. However, using threads requires careful consideration of shared data, thread priorities, and the number of threads. By following these best practices, you can use threads effectively and avoid common pitfalls.
Comentarios