Deep diving into the differences between Python 2 and Python 3
- arpitnearlearn
- Feb 2, 2023
- 2 min read
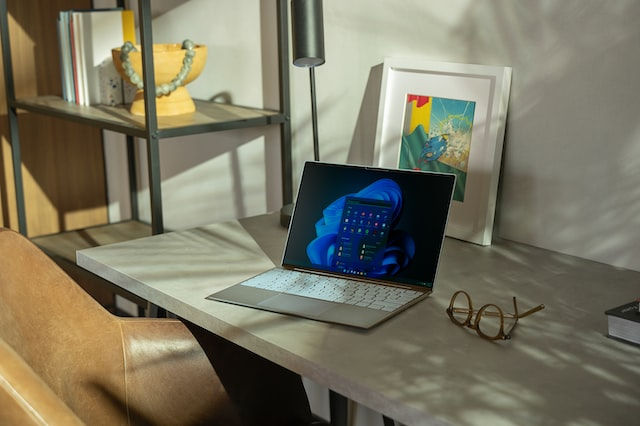
Deep Diving into the Differences between Python 2 and Python 3
Python is a popular, high-level programming language that is widely used for a variety of tasks, from web development to data analysis. However, there are currently two major versions of Python in use: Python 2 and Python 3. In this article, we will take a deep dive into the differences between these two versions of Python, and discuss the factors that should be considered when choosing which version to use for your next project.
Syntax Differences
One of the most noticeable differences between Python 2 and Python 3 is the syntax. Python 3 introduced several changes to the language that are not backward compatible with Python 2. Here are a few examples of these changes:
The print statement in Python 2 is replaced by the print() function in Python 3.
# Python 2
print "Hello, world!"
# Python 3
print("Hello, world!")
The raise statement in Python 2 is replaced by the raise Exception expression in Python 3.
# Python 2
raise ValueError, "Invalid value"
# Python 3
raise ValueError("Invalid value")
The xrange function in Python 2 is replaced by the range function in Python 3.
# Python 2
for i in xrange(10):
print(i)
# Python 3
for i in range(10):
print(i)
If you're looking for training in react native, then you can check out our react native course in Bangalore.
Library Differences
Another major difference between Python 2 and Python 3 is the library. Python 3 introduced several new libraries and modules that are not available in Python 2. Here are a few examples:
The pathlib module was introduced in Python 3.3, which provides an object-oriented approach to working with file and directory paths.
# Python 3
from pathlib import Path
path = Path("/path/to/file.txt")
print(path.name)
The statistics module was introduced in Python 3.4, which provides functions for mathematical statistics.
# Python 3
import statistics
data = [1, 2, 2, 3, 3, 4]
print(statistics.mean(data))
The asyncio module was introduced in Python 3.4, which provides an asynchronous I/O framework for writing concurrent code using coroutines.
# Python 3
import asyncio
async def my_coroutine():
print("Hello, world!")
loop = asyncio.get_event_loop()
loop.run_until_complete(my_coroutine())
Unicode Handling
Python 2 uses ASCII as the default encoding for the str type, which means that it can only handle a limited set of characters. Python 3, on the other hand, uses Unicode as the default encoding for the str type, which means that it can handle a much larger set of characters. This makes Python 3 more suitable for working with internationalisation and localization. If you're looking for training in react native, then you can check out our Python course in Bangalore.
# Python 2
string = "नमस्ते"
print(string)
# Python 3
string = "नमस्ते"
print(string)
Conclusion
Python 2 and Python 3 are both popular versions of Python, but they have several important differences. Python 3 introduced several new features and improvements to the language, such as improved Unicode handling, new libraries and modules, and a new syntax. However, Python 2 is still widely used in many projects and has a large ecosystem of libraries and tools. Near Learn, a technology courses institute in Bangalore, offers courses on both Python 2 and Python 3, which can help developers to understand the differences between the two versions and make an informed decision when choosing which version to use for their next project. It's important to consider the specific requirements of your project and weigh the pros and cons before making a final decision.
Comments