Creating a Full-Stack Web Application with React JS and Python Flask
- arpitnearlearn
- Apr 16, 2023
- 4 min read
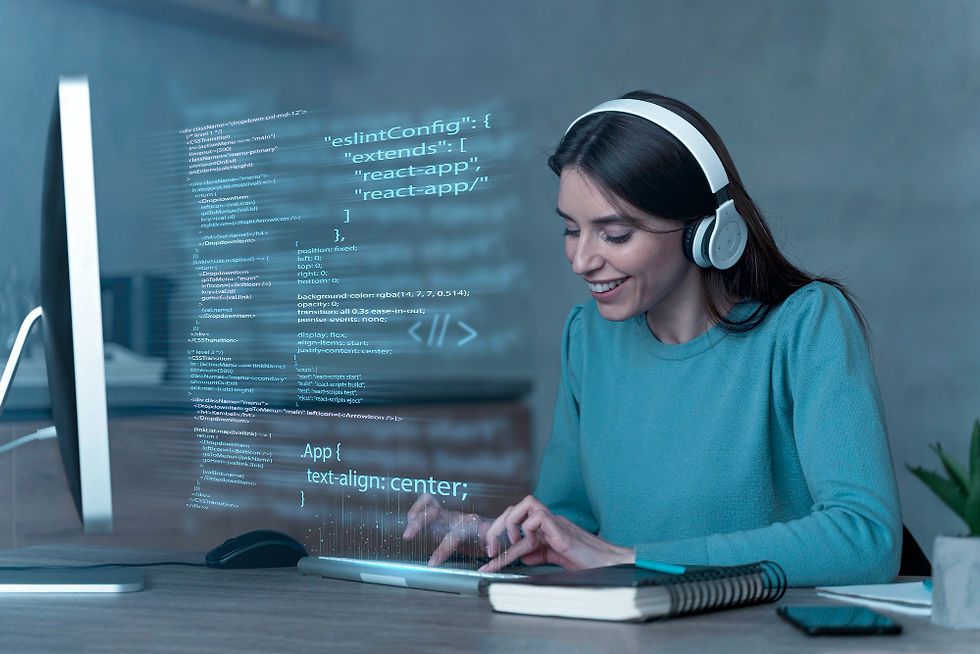
Building a React Native Mobile App with a Python Django API
React Native is a popular framework for building cross-platform mobile apps using JavaScript and React. It allows developers to create high-quality, performant mobile apps that work on both iOS and Android platforms. Python Django is a powerful web framework that can be used to build RESTful APIs that can be used by mobile apps.
In this article, we will walk through the process of building a React Native mobile app that communicates with a Python Django API.
Step 1: Set up the Django API
The first step is to create the Django API that our mobile app will communicate with. To do this, we'll need to create a new Django project and add a new app to it.
First, install Django by running the following command:
pip install django
Next, create a new Django project by running the following command:
django-admin startproject my project
This will create a new Django project called myproject. Next, create a new app called api by running the following command:
bash
cd myproject
python manage.py startapp api
This will create a new app called api inside the myproject project.
Next, open the settings.py file in the myproject folder and add the api app to the INSTALLED_APPS list:
css
INSTALLED_APPS = [ ... 'api',]
Now, open the urls.py file in the myproject folder and add the following code:
php
from django.urls import include, path
urlpatterns = [
path('api/', include('api.urls')),
]
This code creates a new URL pattern that maps to the api app.
Now, create a new file called urls.py in the api folder and add the following code:
python
from django.urls import path
from . import views
urlpatterns = [
path('hello/', views.hello),
]
This code creates a new URL pattern that maps to a new view called hello.
Finally, create a new file called views.py in the api folder and add the following code:
python
from django.http import JsonResponse
def hello(request):
return JsonResponse({'message': 'Hello from Django!'})
This code creates a new view that returns a JSON response with the message "Hello from Django!".
Now, start the Django development server by running the following command:
python manage.py runserver
This will start the development server on http://localhost:8000. If you navigate to http://localhost:8000/api/hello/ in your web browser, you should see the message "Hello from Django!". If you're looking for training in react native, then you can check out our react native course in Bangalore.
Step 2: Set up the React Native app
The next step is to set up the React Native app that will communicate with the Django API. To do this, we'll need to create a new React Native app using the expo-cli command line tool.
First, install the expo-cli tool by running the following command:
npm install -g expo-cli
Next, create a new React Native app by running the following command:
csharp
expo init myapp
This will create a new React Native app called myapp. Choose the "blank" template when prompted.
Next, install the axios library, which we'll use to make API requests, by running the following command:
Copy code
npm install axios
Finally, open the App.js file in the myapp folder and replace the existing code with the following:
python
Copy code
import React, { useEffect, useState } from 'react';
import {
import { StyleSheet, Text, View } from 'react-native';
import axios from 'axios';
export default function App() {
const [message, setMessage] = useState('');
useEffect(() => {
axios.get('http://localhost:8000/api/hello/')
.then(response => {
setMessage(response.data.message);
})
.catch(error => {
console.log(error);
});
}, []);
return (
<View style={styles.container}>
<Text>{message}</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
});
vbnet
Copy code
This code imports the necessary libraries, sets up a state variable called `message`, and makes an API request to the Django server using the `axios` library. The `useEffect` hook is used to make the API request when the component is mounted, and the response is used to update the `message` state variable. Finally, the `message` variable is displayed in the app. If you're looking for training in python, then you can check out our Python course in Bangalore.
Now, start the Expo development server by running the following command:
expo start
vb net
Copy code
This will start the Expo development server on `http://localhost:19002`. You can then run the app on your mobile device using the Expo app or on an emulator using the options provided on the development server page.
Conclusion
In this article, we walked through the process of building a React Native mobile app that communicates with a Python Django API. We created a new Django project and app, set up a new view to return a JSON response, and used the `axios` library to make API requests from our React Native app. By following these steps, you can build powerful mobile apps that communicate with a Python backend using the Django web framework.
In conclusion, building a React Native mobile app that communicates with a Python Django API is a powerful way to create cross-platform mobile applications. React Native offers a simple and efficient way to build mobile applications that work across multiple platforms, while Python and Django provide a flexible and robust backend that can handle complex data processing and business logic. By combining these two technologies, developers can create high-performance mobile apps with a powerful backend API. If you're looking for training in react JS, then you can check out our React JS course in Bangalore.
In this article, we covered the basics of setting up a Python Django API, creating a view to return JSON data, and building a React Native mobile app that communicates with the API. By following these steps, developers can build powerful, cross-platform mobile apps that can handle complex data and business logic. With the power of Python and Django on the backend and React Native on the frontend, the possibilities for mobile app development are endless.
Comments