Creating a Full-Stack Web Application with React JS and Python Flask
- arpitnearlearn
- Feb 21, 2023
- 4 min read
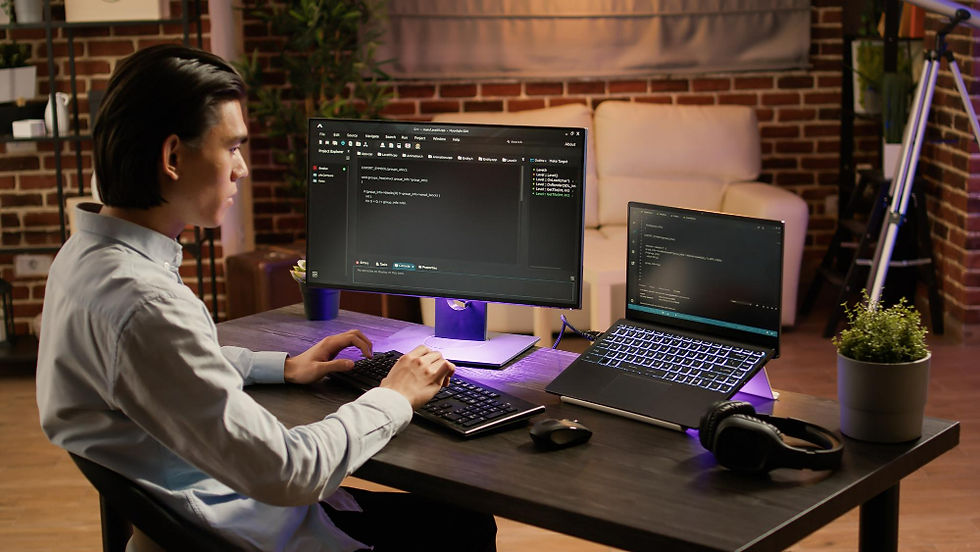
Creating a Full-Stack Web Application with React JS and Python Flask
Full-stack web development is becoming increasingly popular as it allows developers to build both the front-end and back-end of a web application using a single programming language. In this tutorial, we will be building a full-stack web application using React JS for the front-end and Python Flask for the back-end.
Before we get started, make sure you have the following tools and technologies installed:
Node.js and NPM (Node Package Manager)
Python 3 and pip (Python Package Installer)
Once you have these installed, follow the steps below to create your full-stack web application.
Step 1: Create a new React app
First, we need to create a new React app using the create-react-app command. Open your terminal or command prompt and run the following command:
lua
npx create-react-app my-app
This command will create a new React app in a folder called my-app. Once the command completes, navigate to the newly created folder by running:
bash
cd my-app
Step 2: Install and configure Flask
Next, we need to install Flask and set up the back-end of our web application. Open another terminal window and navigate to the same directory where your React app was created. Then, run the following command to create a new folder for the Flask app:
bash
Copy code
mkdir server
cd server
Inside this folder, we need to create a virtual environment and install Flask. Run the following commands:
bash
Copy code
python3 -m venv venv
source venv/bin/activate
pip install flask
This will create a virtual environment and install Flask inside it. Now, create a new file called app.py and add the following code:
python
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/')
def hello():
return jsonify({'message': 'Hello, World!'})
if __name__ == '__main__':
app.run(debug=True)
This code creates a simple Flask app with a single route that returns a JSON response. Run the Flask app by running the following command:
javascript
Copy code
export FLASK_APP=app.py
flask run
This will start the Flask app on http://localhost:5000. If you're looking for training in react native, then you can check out our react native course in Bangalore.
Step 3: Connect React to Flask
Now that we have a working Flask app, let's connect it to our React app. Open the src/App.js file in your React app and replace the contents with the following code:
javascript
Copy code
import React, { useEffect, useState } from 'react';
function App() {
const [message, setMessage] = useState('');
useEffect(() => {
fetch('/api')
.then(response => response.json())
.then(data => setMessage(data.message));
}, []);
return (
<div>
<h1>{message}</h1>
</div>
);
}
export default App;
This code uses the fetch function to make a GET request to /api (which we will define in the Flask app shortly) and displays the message returned in the response.
Now, create a new file called api.py in the server folder and add the following code:
python
from flask import jsonify
from app import app
@app.route('/api')
def api():
return jsonify({'message': 'Hello from Flask!'})
This code defines a new route /api in our Flask app that returns a JSON response. Make sure to import the Flask app instance from app.py.
Finally, restart the Flask app by stopping it (Ctrl+C) and running the flask run command again. Now, start the React app by running the following command in another terminal window:
sql
npm start
This will start the React app on http://localhost:3000.
If everything worked correctly, you should now see the message "Hello from Flask!" displayed in your React app. If you're looking for training in python, then you can check out our Python course in Bangalore.
Step 4: Build the front-end
Now that we have our back-end connected to our front-end, let's build out the rest of the front-end. We'll create a simple form that allows users to enter their name and email address.
Add the following code to App.js:
javascript
Copy code
import React, { useEffect, useState } from 'react';
function App() {
const [message, setMessage] = useState('');
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
fetch('/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
name,
})
})
.then(response => response.json())
.then(data => console.log(data));
};
useEffect(() => {
fetch('/api')
.then(response => response.json())
.then(data => setMessage(data.message));
}, []);
return (
<div>
<h1>{message}</h1>
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" value={name} onChange={e => setName(e.target.value)} />
</label>
<br />
<label>
Email:
<input type="email" value={email} onChange={e => setEmail(e.target.value)} />
</label>
<br />
<button type="submit">Submit</button>
</form>
</div>
);
}
export default App;
This code adds two new state variables name and email, as well as a form with two input fields for those variables. It also adds a handleSubmit function that sends a POST request to /submit (which we will define shortly) with the name and email in the request body. If you're looking for training in react JS, then you can check out our React JS course in Bangalore.
Step 5: Add database functionality
To store the name and email entered by the user, we need to add database functionality to our Flask app. We'll be using SQLite as our database.
First, install the flask_sqlalchemy and flask_cors packages by running the following command:
pip install flask_sqlalchemy flask_cors
Now, create a new file called models.py in the server folder and add the following code:
python
from app import app, db
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(80))
email = db.Column(db.String(120))
def __repr__(self):
return f'<User {self.name}>'
This code defines a new User model with two fields, name and email. It also adds a __repr__ method for easy debugging.
Next, modify app.py to include the following code:
python
Copy code
from flask import jsonify, request
from flask_cors import CORS
from app import app, db
from models import User
CORS(app)
@app.route('/api')
def api():
return jsonify({'message': 'Hello from Flask!'})
@app.route('/submit', methods=['POST'])
def submit():
data = request.get_json()
user = User(name=data['name'], email=data['email'])
db.session.add(user)
db.session.commit()
return jsonify({'message': 'User created!'})
In this article, we have walked through the process of creating a full-stack web application using React JS and Python Flask. We started by setting up a new React app and creating a Flask server to serve the front-end. We then connected the two using CORS and Flask's REST API functionality.
Next, we built out a simple form on the front-end and added database functionality to the Flask server using SQLAlchemy and SQLite. This allowed us to store user data in a database when the form was submitted.
This is just the beginning of what you can do with React JS and Python Flask. With the right tools and techniques, you can build complex, powerful applications that combine the best of both worlds. Whether you're building a simple proof-of-concept or a full-fledged production app, these two technologies offer a powerful combination that can help you achieve your goals.
Comments